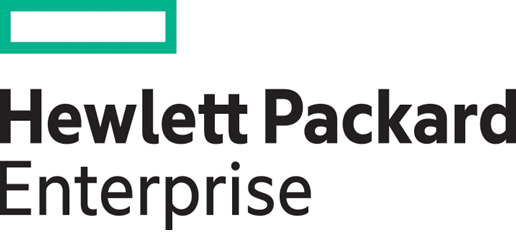
Client Quick Start¶
This section describes the basic functionality of the library for users, who only need a basic client configured for a LegacyRest or Redfish host. For a convenient class for performing higher level functionality or for users unfamiliar with Redfish implementations, see RmcApp Usage.
This Quick Start covers client object creation, a simple call to the API, and a response object.
Scripts of the information provided below are available at: quickstart_redfish.py and quickstart_legacy_rest.py.
For more elaborate examples that use the Redfish API and the python-ilorest-library, see the Examples section.
The python-ilorest-library provides support for communication using both remote using HTTPS and local in-band using CHIF.
Importing the Redfish Library¶
Import the Redfish library.
import redfish
The very first thing that needs to be done for a RESTful request is to create a LegacyRestClient or RedfishClient object.
A RedfishClient is for interacting with a Redfish API, and a LegacyRestClient is for interacting with HPE Legacy Rest API.
For maximum compatibility between iLOs and other vendor hardware that implements Redfish, it is recommended to use the Redfish API whenever possible.
Note
HPE’s Legacy Rest API is available starting in iLO 4 2.00. iLO 4 is Redfish conformant starting with iLO 4 2.30. In iLO 5 and above the iLO RESTful API is Redfish only.
Creating a Remote Object¶
Creating a remote client requires including the iLO hostname or IP address and credentials.
Create a Redfish Object¶
Note
New in version 3.0.0
Creation of a Redfish object instance is done using the RedfishClient class instead of the redfish_client function.
RedfishClient takes as parameters iLO hostname/IP address, username, password, and other optional arguments. For a full list of optional arguments see here.
REST_OBJ = redfish.RedfishClient(base_url=iLO_host, username=login_account, password=login_password)
Create a LegacyRest Object¶
Note
New in version 3.0.0
Creation of a Legacy Rest object instance is done using the LegacyRestClient class instead of the rest_client function.
LegacyRestClient takes as parameters iLO hostname/IP address, username, password, and other optional arguments. For a full list of optional arguments see here.
REST_OBJ = redfish.LegacyRestClient(base_url=iLO_host, username=login_account, password=login_password)
Creating a Local Object¶
Requirements¶
You must be running on a server with iLO and the latest iLO drivers from the SPP.
You will need to download the iLOrest Chif DLL/SO for your corresponding operating system and place it in your working environment path.
By downloading, you agree to the terms and conditions of the Hewlett Packard Enterprise Software License Agreement.
Windows Download: ilorest_chif.dll
Linux Download: ilorest_chif.so
Create a Redfish or LegacyRest Object¶
If you create a LegacyRest or Redfish object without a base_url argument it will automatically create an object configured for a local CHIF connection.
A username and password may be included in object creation if they are required. For most operations in Production security mode no credentials are required locally.
Some reasons credentials may be required locally:
iLO is operating in a higher security mode (Such as HighSecurity).
Some operations require the credentials of an account with a specific privilege to complete (Even in Production security mode).
REST_OBJ = redfish.RedfishClient()
Create a login session¶
Next the rest object’s login method is called to initiate a rest session. The login method takes the credentials specified in the REST object we created. Login also takes an authentication type optional parameter, if the argument is not included the Client will choose an authentication type for you based on the arguments given with session being the default.
For session authentication, a session key is generated through a rest request, the default for username and password arguments.
For basic authentication, the username and password is base64 encoded and sent in a header for each request. For security, session authentication is preferred.
REST_OBJ.login(auth="session")
Remember to call logout method once the session is completed to free up sessions.
Perform your first RESTful API GET operation¶
The following example performs a GET operation on the systems resource (/redfish/v1/systems/1) using the HPE RESTful API for iLO. It does an HTTP GET request on the iLO SSL(HTTPS) port (typically 443 but the iLO can be configured to use another port as well). The interface is not available over open HTTP (port 80), so SSL handshake must be used.
Perform a HTTP GET request by using the get method.
>>> response = REST_OBJ.get('/redfish/v1/systems/1')
Response Object¶
A Response object called response is now available for us to inspect.
The status property returns the HTTP response to our request:
>>> response.status
200
The dict property returns the response json body as a Python dictionary:
>>> response.dict
The getheaders() method returns a dictionary of all http response headers:
>>> response.getheaders()
{'Transfer-Encoding': 'chunked', 'X_HP-CHRP-Service-Version': '1.0.3', 'ETag': 'W/"0129EA9F"',
'Link': '</redfish/v1/SchemaStore/en/ComputerSystem.json>;
rel=describedby', 'Allow': 'GET, HEAD, POST, PATCH',
'Cache-Control': 'no-cache', 'Date': 'Tue, 06 Aug 2019 19:42:26 GMT',
'OData-Version': '4.0', 'X-Frame-Options': 'sameorigin', 'Content-type': 'application/json; charset=utf-8'}
You can also print the Response object directly:
>>> sys.stdout.write("%s\n" % response)
Response status:
200
Response header:
Transfer-Encoding chunkedX_HP-CHRP-Service-Version 1.0.3ETag W/”E4BDA463”Link </redfish/v1/SchemaStore/en/ComputerSystem.json>; rel=describedbyAllow GET, HEAD, POST, PATCHCache-Control no-cacheDate Thu, 01 Aug 2019 19:13:17 GMTOData-Version 4.0X-Frame-Options sameoriginContent-type application/json; charset=utf-8
The formated Response body (Truncated for size):
{
"@odata.context": "/redfish/v1/$metadata#ComputerSystem.ComputerSystem",
"@odata.etag": "W/\"E4BDA463\"",
"@odata.id": "/redfish/v1/Systems/1",
"@odata.type": "#ComputerSystem.v1_4_0.ComputerSystem",
"Id": "1",
"Actions": {
"#ComputerSystem.Reset": {
"ResetType@Redfish.AllowableValues": [
"On",
"ForceOff",
"ForceRestart",
"Nmi",
"PushPowerButton"
],
"target": "/redfish/v1/Systems/1/Actions/ComputerSystem.Reset"
}
},
"AssetTag": "",
"Bios": {
"@odata.id": "/redfish/v1/systems/1/bios"
}
}
A full description of the Response Object is available in the reference here.
Other HTTP Requests¶
Other HTTP Requests HEAD, PATCH, POST, DELETE, and PUT are just as simple to use as GET.
>>> response = REST_OBJ.head('/redfish/v1/systems/1')
>>> response = REST_OBJ.patch('/redfish/v1/systems/1', {'AssetTag': 'newtag'})
>>> response = REST_OBJ.post('/redfish/v1/Systems/1/Actions/ComputerSystem.Reset/', {'ResetType': 'ForceRestart'})
>>> response = REST_OBJ.delete(REST_OBJ.session_location)
>>> response = REST_OBJ.put('<uri>', 'data')
Close the login session¶
Logout of the current session. This is only required for session based authentication.
REST_OBJ.logout()
Continued Reading¶
That’s it! You’re ready to perform some Redfish requests! If you are looking for more advanced topics like setting timeouts, more detail on the response object, or configuration of the Redfish or LegacyRest objects check out the Advanced Usage section.